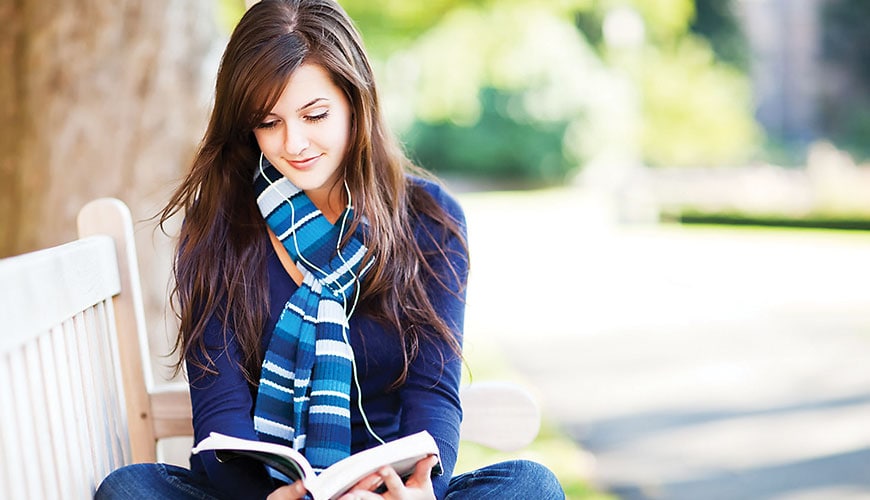
MongoDB is a document database. It stores data in a type of JSON format called BSON.
If you are unfamiliar with JSON, check out our JSON tutorial.
A record in MongoDB is a document, which is a data structure composed of key value pairs similar to the structure of JSON objects.
A MongoDB Document
Records in a MongoDB database are called documents, and the field values may include numbers, strings, booleans, arrays, or even nested documents.
Example Document
{
title: "Post Title 1",
body: "Body of post.",
category: "News",
likes: 1,
tags: ["news", "events"],
date: Date()
}
Learning by Examples
Our “Show MongoDB” tool makes it easy to demonstrate MongoDB. It shows both the code and the result.
Example
Find all documents that have a category of “news”.
db.posts.find( {category: "News"} )
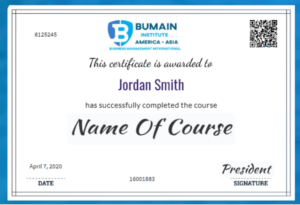
Curriculum
- 16 Sections
- 25 Lessons
- 10 Weeks
- MongoDB Getting StartedMongoDB is a document database and can be installed locally or hosted in the cloud.1
- MongoDB Query APIThe MongoDB Query API is the way you will interact with your data. The MongoDB Query API can be used two ways: CRUD Operations Aggregation Pipelines1
- MongoDB mongosh Create DatabaseAfter connecting to your database using mongosh, you can see which database you are using by typing db in your terminal. If you have used the connection string provided from the MongoDB Atlas dashboard, you should be connected to the myFirstDatabase database.1
- MongoDB mongosh InsertThere are 2 methods to insert documents into a MongoDB database. insertOne() To insert a single document, use the insertOne() method. This method inserts a single object into the database.1
- MongoDB mongosh FindThere are 2 methods to find and select data from a MongoDB collection, find() and findOne().1
- MongoDB mongosh UpdateTo update an existing document we can use the updateOne() or updateMany() methods. The first parameter is a query object to define which document or documents should be updated. The second parameter is an object defining the update1
- MongoDB mongosh DeleteWe can delete documents by using the methods deleteOne() or deleteMany(). These methods accept a query object. The matching documents will be deleted.1
- MongoDB Query OperatorsThere are many query operators that can be used to compare and reference document fields.1
- MongoDB Update OperatorsThere are many update operators that can be used during document updates.1
- MongoDB Aggregation Pipelinesggregation operations allow you to group, sort, perform calculations, analyze data, and much more. Aggregation pipelines can have one or more "stages". The order of these stages are important. Each stage acts upon the results of the previous stage.10
- Indexing & SearchMongoDB Atlas comes with a full-text search engine that can be used to search for documents in a collection.1
- MongoDB Schema ValidationBy default MongoDB has a flexible schema. This means that there is no strict schema validation set up initially. Schema validation rules can be created in order to ensure that all documents a collection share a similar structure.1
- MongoDB Data APIThe MongoDB Data API can be used to query and update data in a MongoDB database without the need for language specific drivers. Language drivers should be used when possible, but the MongoDB Data API comes in handy when drivers are not available or drivers are overkill for the application.1
- MongoDB DriversThe MongoDB Shell (mongosh) is great, but generally you will need to use MongoDB in your application. To do this, MongoDB has many language drivers. The language drivers allow you to interact with your MongoDB database using the methods you've learned so far in `mongosh` but directly in your application.1
- MongoDB Node.js Database InteractionFor this tutorial, we will use a MongoDB Atlas database. If you don't already have a MongoDB Atlas account, you can create one for free at MongoDB Atlas. We will also use the "sample_mflix" database loaded from our sample data in the Intro to Aggregations section.1
- MongoDB ChartsMongoDB Charts lets you visualize your data in a simple, intuitive way.1